简介
Gravity: SCD41二氧化碳传感器,搭载了Sensirion公司新推出SCD41微型二氧化碳传感器传感器。该传感器基于光声传感原理以及Sensirion的PASens®和CMOSens® 专利技术,体积小巧,性能卓越。集成性价比极佳的湿度和温度传感器,实现片上信号补偿,并具备额外湿度和温度输出。
SCD41通过监测CO2分子吸收的能量测量CO2浓度。当发射红外脉冲时,CO2分子周期性的吸收红外线,这会引起额外的分子振动,从而会在腔内产生压力波。CO2浓度越高,吸收的光就越多,因此声波的振幅越大,通过内部麦克风测量、计算得出CO2浓度。
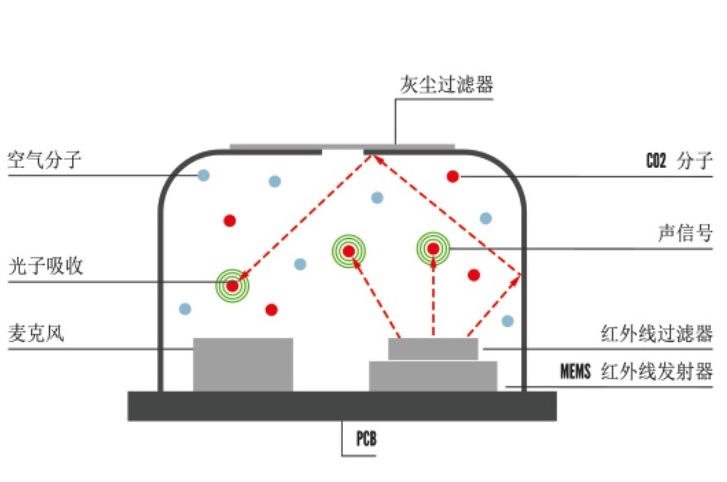
特性
- CO2、温度、湿度三合一
- 体积小巧,仅32*27*8mm
- 功耗低,平均电流<4mA
应用场景
- 室内CO2浓度监测
- 大棚植物环境监测
- 新风系统环境检测
技术规格
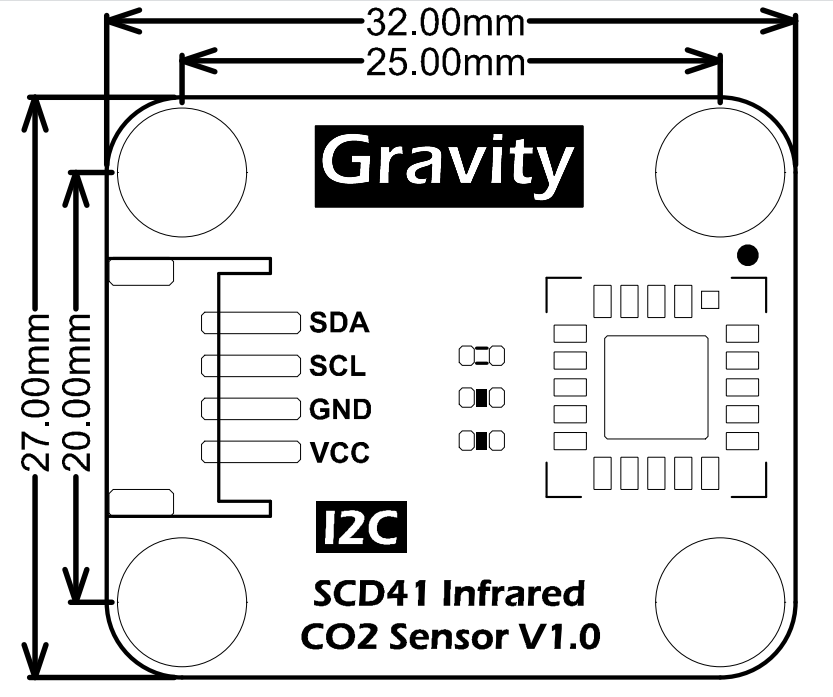
- 供电电压:3.3V~5V
- 平均工作电流:<4mA
- I2C地址:0x62
- 产品尺寸:32*27*8mm
二氧化碳
- 精确度:±(40 ppm + 5% MV)
- 测量范围:400 - 5000 ppm
- 响应时间:60S
湿度
- 典型相对湿度精度:6%RH
- 相对湿度测量范围:0 ~ 95%RH
- 响应时间:120S
温度
- 典型温度精度:0.8℃
- 温度测量范围:-10 ~ 60℃
- 响应时间:120S
引脚说明
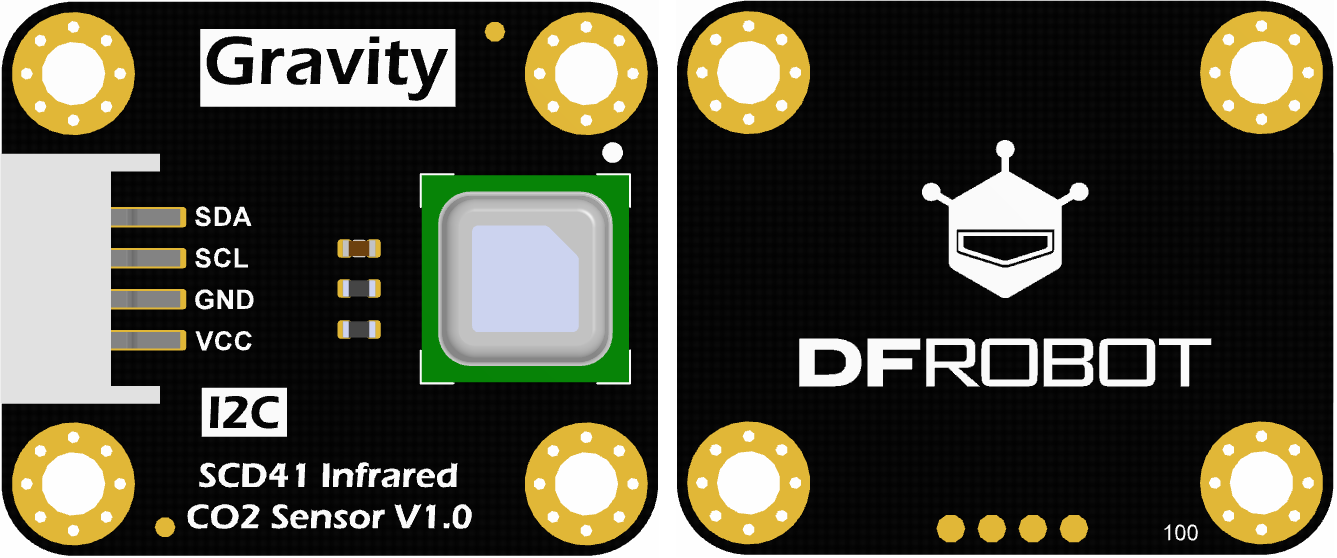
序号 | 丝印 | 功能描述 |
---|---|---|
1 | VCC | 电源正极 |
2 | GND | 电源负极 |
3 | SCL | I2C时钟线 |
4 | SDA | I2C数据线 |
Arduino使用教程
准备
- 硬件
- 1 x Arduino UNO控制板
- 1 x Gravity:SCD41二氧化碳传感器
- 若干 杜邦线
- 软件
- Arduino IDE, 点击下载Arduino IDE
- SCD41库文件和示例程序
关于如何安装库文件,点击链接
接线图
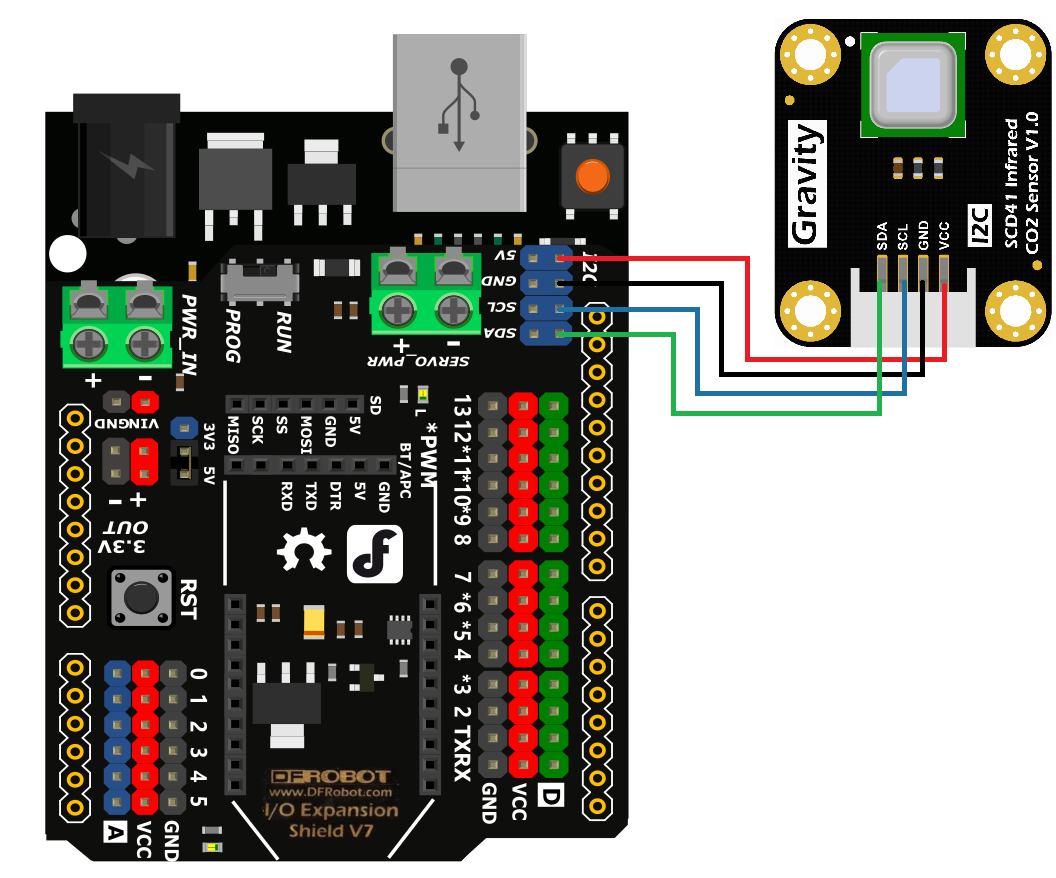
样例代码1 - 周期读取
循环读取SCD41数据
/*!
* @file periodMeasure.ino
* @brief This sample shows how to configure period measurement mode, compensation and calibration.
* @note The actual compensation and calibration parameter should be changed according to the operating environment
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author [qsjhyy](yihuan.huang@dfrobot.com)
* @version V1.0
* @date 2022-05-11
* @url https://github.com/DFRobot/DFRobot_SCD4X
*/
#include <DFRobot_SCD4X.h>
/**
* @brief Constructor
* @param pWire - Wire object is defined in Wire.h, so just use &Wire and the methods in Wire can be pointed to and used
* @param i2cAddr - SCD4X I2C address.
*/
DFRobot_SCD4X SCD4X(&Wire, /*i2cAddr = */SCD4X_I2C_ADDR);
void setup(void)
{
Serial.begin(115200);
// Init the sensor
while( !SCD4X.begin() ){
Serial.println("Communication with device failed, please check connection");
delay(3000);
}
Serial.println("Begin ok!");
/**
* @brief set periodic measurement mode
* @param mode - periodic measurement mode:
* @n SCD4X_START_PERIODIC_MEASURE : start periodic measurement, signal update interval is 5 seconds.
* @n SCD4X_STOP_PERIODIC_MEASURE : stop periodic measurement command
* @n SCD4X_START_LOW_POWER_MEASURE : start low power periodic measurement, signal update interval is approximately 30 seconds.
* @return None
* @note The measurement mode must be disabled when configuring the sensor; after giving the stop_periodic_measurement command, the sensor needs to wait 500ms before responding to other commands.
*/
SCD4X.enablePeriodMeasure(SCD4X_STOP_PERIODIC_MEASURE);
/**
* @brief set temperature offset
* @details T(offset_actual) = T(SCD4X) - T(reference) + T(offset_previous)
* @n T(offset_actual): the calculated actual temperature offset that is required
* @n T(SCD4X): the temperature measured by the sensor (wait for a period of time to get steady readings)
* @n T(reference): the standard reference value of the current ambient temperature
* @n T(offset_previous): the previously set temperature offset
* @n For example : 32(T(SCD4X)) - 30(T(reference)) + 2(T(offset_previous)) = 4(T(offset_actual))
* @param tempComp - temperature offset value, unit ℃
* @return None
* @note When executing the command, the sensor can't be in period measurement mode
*/
SCD4X.setTempComp(4.0);
/**
* @brief get temperature offset
* @return The current set temp compensation value, unit ℃
* @note When executing the command, the sensor can't be in period measurement mode
*/
float temp = 0;
temp = SCD4X.getTempComp();
Serial.print("The current temperature compensation value : ");
Serial.print(temp);
Serial.println(" C");
/**
* @brief set sensor altitude
* @param altitude - the current ambient altitude, unit m
* @return None
* @note When executing the command, the sensor can't be in period measurement mode
*/
SCD4X.setSensorAltitude(540);
/**
* @brief get sensor altitude
* @return The current set ambient altitude, unit m
* @note When executing the command, the sensor can't be in period measurement mode
*/
uint16_t altitude = 0;
altitude = SCD4X.getSensorAltitude();
Serial.print("Set the current environment altitude : ");
Serial.print(altitude);
Serial.println(" m");
/**
* @brief set automatic self calibration enabled
* @param mode - automatic self-calibration mode:
* @n true : enable automatic self-calibration
* @n false : disable automatic self-calibration
* @return None
* @note When executing the command, the sensor can't be in period measurement mode
*/
// SCD4X.setAutoCalibMode(true);
/**
* @brief get automatic self calibration enabled
* @return Automatic self-calibration mode:
* @n true : enable automatic self-calibration
* @n false : disable automatic self-calibration
* @note When executing the command, the sensor can't be in period measurement mode
*/
// if(SCD4X.getAutoCalibMode()) {
// Serial.println("Automatic calibration on!");
// } else {
// Serial.println("Automatic calibration off!");
// }
/**
* @brief persist settings
* @details Configuration settings such as the temperature offset, sensor altitude and the ASC enabled/disabled
* @n parameter are by default stored in the volatile memory (RAM) only and will be lost after a power-cycle.
* @return None
* @note To avoid unnecessary wear of the EEPROM, the persist_settings command should only be sent
* @n when persistence is required and if actual changes to the configuration have been made.
* @n The EEPROM is guaranteed to endure at least 2000 write cycles before failure.
* @note Command execution time : 800 ms
* @n When executing the command, the sensor can't be in period measurement mode
*/
// SCD4X.persistSettings();
/**
* @brief reinit reinit
* @details The reinit command reinitializes the sensor by reloading user settings from EEPROM.
* @return None
* @note Before sending the reinit command, the stop measurement command must be issued.
* @n If the reinit command does not trigger the desired re-initialization,
* @n a power-cycle should be applied to the SCD4x.
* @n Command execution time : 20 ms
* @n When executing the command, the sensor can't be in period measurement mode
*/
//SCD4X.moduleReinit();
/**
* @brief set periodic measurement mode
* @param mode - periodic measurement mode:
* @n SCD4X_START_PERIODIC_MEASURE : start periodic measurement, signal update interval is 5 seconds.
* @n SCD4X_STOP_PERIODIC_MEASURE : stop periodic measurement command
* @n SCD4X_START_LOW_POWER_MEASURE : start low power periodic measurement, signal update interval is approximately 30 seconds.
* @return None
* @note The measurement mode must be disabled when changing the sensor settings; after giving the stop_periodic_measurement command, the sensor needs to wait 500ms before responding to other commands.
*/
SCD4X.enablePeriodMeasure(SCD4X_START_PERIODIC_MEASURE);
Serial.println();
}
void loop()
{
/**
* @brief get data ready status
* @return data ready status:
* @n true : data ready
* @n false : data not ready
*/
if(SCD4X.getDataReadyStatus()) {
/**
* @brief set ambient pressure
* @param ambientPressure - the current ambient pressure, unit Pa
* @return None
*/
// SCD4X.setAmbientPressure(96000);
/**
* @brief Read the measured data
* @param data - sSensorMeasurement_t, the values measured by the sensor, including CO2 concentration (ppm), temperature (℃) and humidity (RH)
* @n typedef struct {
* @n uint16_t CO2ppm;
* @n float temp;
* @n float humidity;
* @n } sSensorMeasurement_t;
* @return None
* @note CO2 measurement range: 0~40000 ppm; temperature measurement range: -10~60 ℃; humidity measurement range: 0~100 %RH.
*/
DFRobot_SCD4X::sSensorMeasurement_t data;
SCD4X.readMeasurement(&data);
Serial.print("Carbon dioxide concentration : ");
Serial.print(data.CO2ppm);
Serial.println(" ppm");
Serial.print("Environment temperature : ");
Serial.print(data.temp);
Serial.println(" C");
Serial.print("Relative humidity : ");
Serial.print(data.humidity);
Serial.println(" RH");
Serial.println();
}
delay(1000);
}
结果
样例代码2 - 单次测量
休眠醒来后读取数据,然后再休眠
/*!
* @file singleShotMeasure.ino
* @brief This sample shows how to set single measurement mode, perform reset operation, and set sleep and wake-up mode.
* @details Get 6 data from single measurement, take the average value, print it, enter sleep mode, wake up the sensor after 5 minutes, and repeat the above measurement process
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author [qsjhyy](yihuan.huang@dfrobot.com)
* @version V1.0
* @date 2022-05-11
* @url https://github.com/DFRobot/DFRobot_SCD4X
*/
#include <DFRobot_SCD4X.h>
/**
* @brief Constructor
* @param pWire - Wire object is defined in Wire.h, so just use &Wire and the methods in Wire can be pointed to and used
* @param i2cAddr - SCD4X I2C address.
*/
DFRobot_SCD4X SCD4X(&Wire, /*i2cAddr = */SCD4X_I2C_ADDR);
void setup(void)
{
Serial.begin(115200);
// Init the sensor
while( !SCD4X.begin() ){
Serial.println("Communication with device failed, please check connection");
delay(3000);
}
Serial.println("Begin ok!");
/**
* @brief set periodic measurement mode
* @param mode - periodic measurement mode:
* @n SCD4X_START_PERIODIC_MEASURE : start periodic measurement, signal update interval is 5 seconds.
* @n SCD4X_STOP_PERIODIC_MEASURE : stop periodic measurement command
* @n SCD4X_START_LOW_POWER_MEASURE : start low power periodic measurement, signal update interval is approximately 30 seconds.
* @return None
* @note The measurement mode must be disabled when configuring the sensor; after giving the stop_periodic_measurement command, the sensor needs to wait 500ms before responding to other commands.
*/
SCD4X.enablePeriodMeasure(SCD4X_STOP_PERIODIC_MEASURE);
/**
* @brief perform self test
* @details The perform_self_test feature can be used as an end-of-line test to check sensor
* @n functionality and the customer power supply to the sensor.
* @return module status:
* @n 0 : no malfunction detected
* @n other : malfunction detected
* @note Command execution time : 10000 ms
* @n When executing the command, the sensor can't be in period measurement mode.
*/
if(0 != SCD4X.performSelfTest()) {
Serial.println("Malfunction detected!");
}
Serial.println();
}
void loop()
{
/**
* @brief Set the sensor as sleep or wake-up mode (SCD41 only)
* @param mode - Sleep and wake-up mode:
* @n SCD4X_POWER_DOWN : Put the sensor from idle to sleep to reduce current consumption.
* @n SCD4X_WAKE_UP : Wake up the sensor from sleep mode into idle mode.
* @return None
* @note Note that the SCD4x does not acknowledge the wake_up command. Command execution time : 20 ms
* @n When executing the command, the sensor can't be in period measurement mode.
*/
Serial.print("Waking sensor...");
SCD4X.setSleepMode(SCD4X_WAKE_UP);
DFRobot_SCD4X::sSensorMeasurement_t data[6];
memset(data, 0, sizeof(data));
uint32_t averageCO2ppm=0;
float averageTemp=0.0, averageHumidity=0.0;
Serial.print("Measuring...");
for(uint8_t i=0; i<6; i++) {
/**
* @brief measure single shot(SCD41 only)
* @details On-demand measurement of CO2 concentration, relative humidity and temperature.
* @n Get the measured data through readMeasurement(sSensorMeasurement_t data) interface
* @param mode - Single-measurement mode:
* @n SCD4X_MEASURE_SINGLE_SHOT : On-demand measurement of CO2 concentration, relative humidity and temperature.
* @n Max command duration 5000 [ms].
* @n SCD4X_MEASURE_SINGLE_SHOT_RHT_ONLY : On-demand measurement of relative humidity and temperature only.
* @n Max command duration 50 [ms].
* @note In SCD4X_MEASURE_SINGLE_SHOT_RHT_ONLY mode, CO2 output is returned as 0 ppm.
* @return None
* @note When executing the command, the sensor can't be in period measurement mode.
*/
SCD4X.measureSingleShot(SCD4X_MEASURE_SINGLE_SHOT);
/**
* @brief get data ready status
* @return data ready status:
* @n true : data ready
* @n false : data not ready
*/
while(!SCD4X.getDataReadyStatus()) {
delay(100);
}
/**
* @brief Read the measured data
* @param data - sSensorMeasurement_t, the values measured by the sensor, including CO2 concentration (ppm), temperature (℃) and humidity (RH)
* @n typedef struct {
* @n uint16_t CO2ppm;
* @n float temp;
* @n float humidity;
* @n } sSensorMeasurement_t;
* @return None
* @note CO2 measurement range: 0~40000 ppm; temperature measurement range: -10~60 ℃; humidity measurement range: 0~100 %RH.
*/
SCD4X.readMeasurement(&data[i]);
if(0 != i) { // Discard the first set of data, because the chip datasheet indicates the first reading obtained after waking up is invalid
averageCO2ppm += data[i].CO2ppm;
averageTemp += data[i].temp;
averageHumidity += data[i].humidity;
}
Serial.print(i);
}
Serial.print("\nCarbon dioxide concentration : ");
Serial.print(averageCO2ppm / 5);
Serial.println(" ppm");
Serial.print("Environment temperature : ");
Serial.print(averageTemp / 5);
Serial.println(" C");
Serial.print("Relative humidity : ");
Serial.print(averageHumidity / 5);
Serial.println(" RH\n");
// Put the sensor from idle to sleep to reduce current consumption.
Serial.print("Sleeping sensor...");
SCD4X.setSleepMode(SCD4X_POWER_DOWN);
delay(300000); // Wake up the sensor after 5 minutes, and repeat the above measurement process
}
结果
主要API接口函数列表
/**
* @fn enablePeriodMeasure
* @brief set periodic measurement mode
* @param mode - periodic measurement mode:
* @n SCD4X_START_PERIODIC_MEASURE : start periodic measurement, signal update interval is 5 seconds.
* @n SCD4X_STOP_PERIODIC_MEASURE : stop periodic measurement command
* @n SCD4X_START_LOW_POWER_MEASURE : start low power periodic measurement, signal update interval is approximately 30 seconds.
* @return None
* @note 在对传感器进行设置的时候,必须停止测量模式; 且在发出stop_periodic_measurement命令后,传感器等待500ms后才响应其他命令。
*/
void enablePeriodMeasure(uint16_t mode);
/**
* @fn readMeasurement
* @brief 读取测量数据
* @param data - sSensorMeasurement_t, 传感器测量值,包含CO2浓度(ppm)、温度(C)、湿度(RH)
* @return None
* @note CO2测量范围: 0~40000 ppm; 温度测量范围: -10~60 C; 湿度测量范围: 0~100 %RH.
*/
void readMeasurement(sSensorMeasurement_t * data);
/**
* @fn getDataReadyStatus
* @brief get data ready status
* @return data ready status:
* @n true : data ready
* @n false : data not ready
*/
bool getDataReadyStatus(void);
树莓派使用教程
准备
-
硬件
- 树莓派4代B型(或类似)主控板 x 1
- Gravity:SCD41二氧化碳传感器 x 1
- 若干杜邦线 x 1
-
软件
接线图
- 将模块与树莓派按照连线图相连。I2C地址为0x62
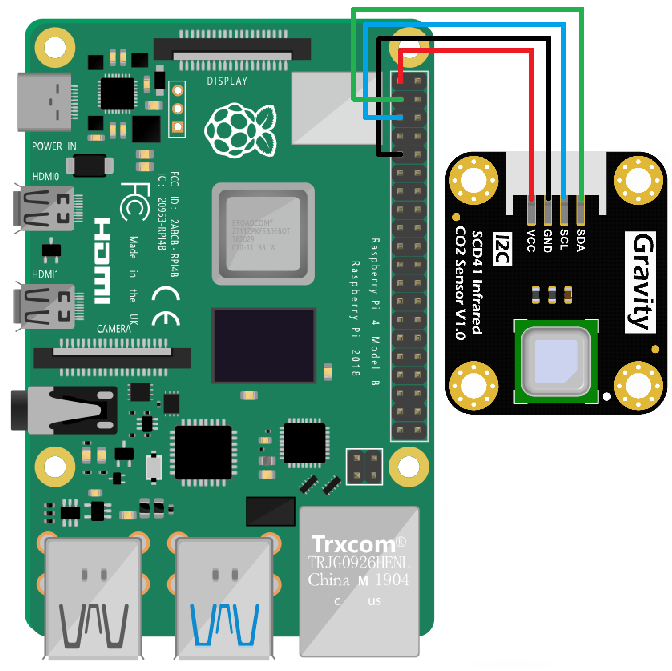
安装驱动
-
启动树莓派的I2C接口。如已开启,可跳过该步骤。
打开终端(Terminal),键入如下指令,并回车:
pi@raspberrypi:~ $ sudo raspi-config
然后用上下键选择“ 5 Interfacing Options ”, 按回车进入,选择 “ P5 I2C ”, 按回车确认“ YES ”即可。重启树莓派主控板。 -
安装Python依赖库与git,树莓派需要联网。如已安装,可跳过该步骤。
在终端中,依次键入如下指令,并回车:
pi@raspberrypi:~ $ sudo apt-get update
pi@raspberrypi:~ $ sudo apt-get install build-essential python-dev python-smbus git
-
下载DFRobot_SCD4X驱动库。在终端中,依次键入如下指令,并回车:
pi@raspberrypi:~ $ cd Desktop/
pi@raspberrypi:~/Desktop $ git clone https://github.com/dfrobot/DFRobot_SCD4X
样例代码
样例代码1-连续测量(period_measure.py)
- 在终端中,键入如下指令并回车,运行样例代码:
pi@raspberrypi:~/Desktop $ cd DFRobot_SCD4X/python/raspberrypi/examples/
pi@raspberrypi:~/Desktop/DFRobot_SCD4X/python/raspberrypi/examples/ $ python period_measure.py
样例代码2-单次测量(single_shot_measure.py)
- 在终端中,键入如下指令并回车,运行样例代码:
pi@raspberrypi:~/Desktop $ cd DFRobot_SCD4X/python/raspberrypi/examples/
pi@raspberrypi:~/Desktop/DFRobot_SCD4X/python/raspberrypi/examples/ $ python single_shot_measure.py
Mind+使用教程
说明
使用Mind+1.7.3及以上版本,打开扩展,在用户库中粘贴库链接加载用户库。
- scd4xco2模块Mind+库:https://gitee.com/dfjoannali/ext-scd4xco2
程序示例
-
Arduino模式uno:
-
Python模式行空板:
常见问题
还没有客户对此产品有任何问题,欢迎通过qq或者论坛联系我们!
更多问题及有趣的应用,可以 访问论坛 进行查阅或发帖。