简介
VL6180X是意法半导体的一款ToF激光测距传感器,可测量5-100mm的距离,但在良好的环境下测量距离可达200mm。VL6180X搭载了意法半导体FlightSense ™技术,可以精确的测量距离,与目标反射率无关。同时VL6180X还搭载了环境光传感器,可以测量出环境光数据。
特性
- 可同时测量距离和环境光
- VCSEL光源
- 抗干扰性强
应用场景
- 短距离测量应用
- 机器人障碍物检测
- 距离触发开关
技术规格
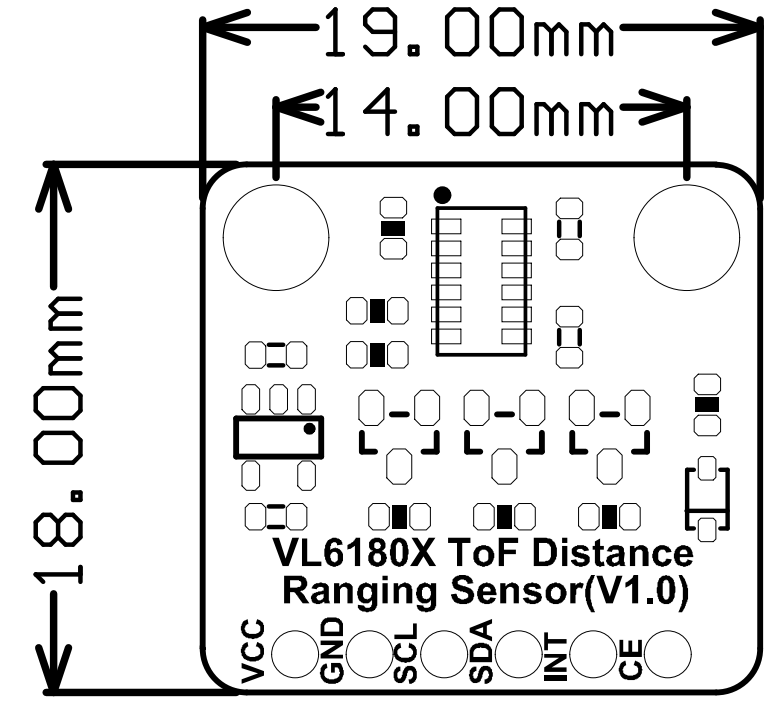
- 供电电压:3.3V~5V
- 工作电流:<5mA
- 测量距离:5-100mm
- 发射器:940 nm不可见激光(VCSEL)
- I2C地址:0x29
- 工作温度范围:-20℃~85℃
- 产品尺寸:19*18mm
引脚说明
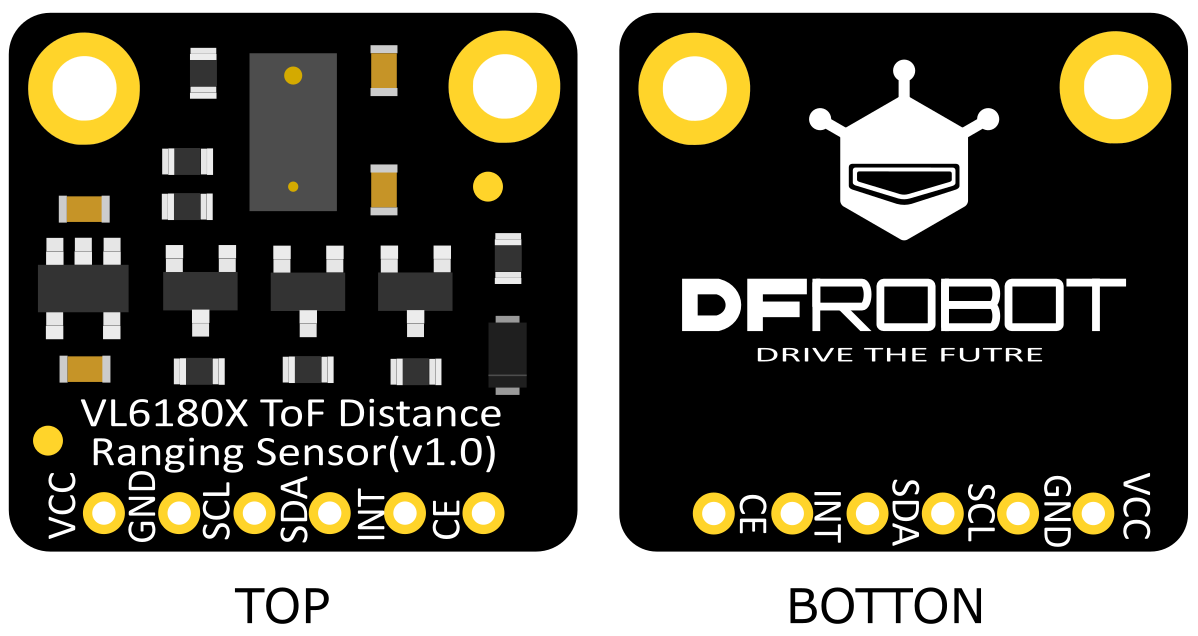
序号 | 丝印 | 功能描述 |
---|---|---|
1 | VCC | 电源正极 |
2 | GND | 电源负极 |
3 | SCL | I2C时钟线 |
4 | SDA | I2C数据线 |
5 | INT | 指示传感器数据准备就绪 |
6 | CE | 使能引脚,低电平关闭传感器 |
使用教程
准备
- 硬件
- 1 x Arduino UNO控制板
- 1 x ToF激光测距传感器
- 若干 杜邦线
- 软件
- Arduino IDE, 点击下载Arduino IDE
- VL6180X库文件和示例程序
关于如何安装库文件,点击链接
- 主要API接口函数列表
/** param:mode default: VL6180X_SINGEL
VL6180X_SINGEL 0x00 A single measurement of ALS and range
VL6180X_CONTINUOUS_RANGE 0x01 Continuous measuring range
VL6180X_CONTINUOUS_ALS 0x02 Continuous measuring ALS
VL6180X_INTERLEAVED_MODE 0x03 Continuous cross measurement of ALS and range
*/
VL6180X.setMode(VL6180X_INTERLEAVED_MODE);
/**
* @brief Obtain ambient light data
* @return Measured ambient light data
*/
float getALSValue();
/**
* @brief Obtain range data
* @return Measured range data
*/
uint8_t getRangeVlaue();
接线图
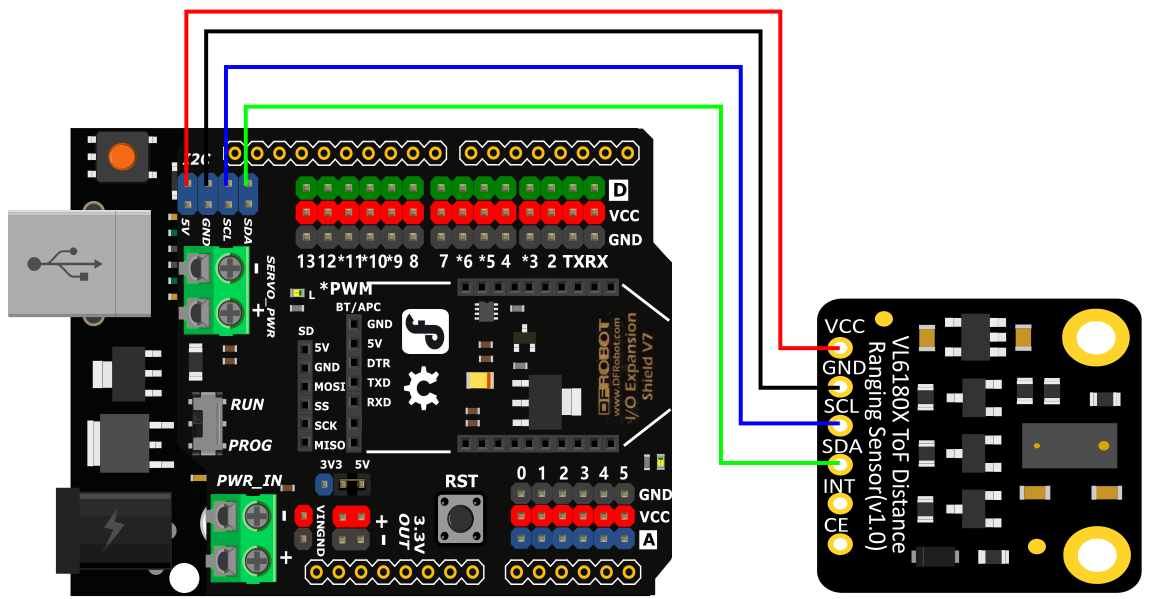
样例代码1 - 通过轮询的方式读取数据
烧录代码,打开串口,串口打印出测量的环境光数据和距离。
/**!
* @file pollMeasurement.ino
* @brief Measures absolute range from 0 to above 10 cm
* @n Measurement of ambient light data
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [yangfeng]<feng.yang@dfrobot.com>
* @version V1.0
* @date 2021-02-08
* @get from https://www.dfrobot.com
* @url https://github.com/DFRobot/DFRobot_VL6180X
*/
#include <DFRobot_VL6180X.h>
//修改I2C地址,掉电后失效
//DFRobot_VL6180X VL6180X(/* iicAddr */0x29,/* TwoWire * */&Wire);
DFRobot_VL6180X VL6180X;
void setup() {
Serial.begin(9600);
while(!(VL6180X.begin())){
Serial.println("Please check that the IIC device is properly connected!");
delay(1000);
}
/*更改IIC地址*/
//VL6180X.setIICAddr(0x29);
}
void loop() {
/*轮询的测量环境光数据*/
float lux = VL6180X.alsPoLLMeasurement();
String str ="ALS: "+String(lux)+" lux";
Serial.println(str);
delay(1000);
/*轮询的测量距离*/
uint8_t range = VL6180X.rangePollMeasurement();
/*获得范围值的判断结果*/
uint8_t status = VL6180X.getRangeResult();
String str1 = "Range: "+String(range) + " mm";
switch(status){
case VL6180X_NO_ERR:
Serial.println(str1);
break;
case VL6180X_EARLY_CONV_ERR:
Serial.println("RANGE ERR: ECE check failed !");
break;
case VL6180X_MAX_CONV_ERR:
Serial.println("RANGE ERR: System did not converge before the specified max!");
break;
case VL6180X_IGNORE_ERR:
Serial.println("RANGE ERR: Ignore threshold check failed !");
break;
case VL6180X_MAX_S_N_ERR:
Serial.println("RANGE ERR: Measurement invalidated!");
break;
case VL6180X_RAW_Range_UNDERFLOW_ERR:
Serial.println("RANGE ERR: RESULT_RANGE_RAW < 0!");
break;
case VL6180X_RAW_Range_OVERFLOW_ERR:
Serial.println("RESULT_RANGE_RAW is out of range !");
break;
case VL6180X_Range_UNDERFLOW_ERR:
Serial.println("RANGE ERR: RESULT__RANGE_VAL < 0 !");
break;
case VL6180X_Range_OVERFLOW_ERR:
Serial.println("RANGE ERR: RESULT__RANGE_VAL is out of range !");
break;
default:
Serial.println("RANGE ERR: Systerm err!");
break;
}
delay(1000);
}
结果
样例代码2 - 通过中断的方式读取数据
烧录代码,打开串口,串口打印出测量的环境光数据和距离。
/**
* @file interleaveMode.ino
* @brief 本示例介绍了在交叉测量模式下的进行中断方式的测量光照数据以及范围数据。
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [yangfeng]<feng.yang@dfrobot.com>
* @version V1.0
* @date 2021-03-08
* @get from https://www.dfrobot.com
* @url https://github.com/DFRobot/DFRobot_VL6180X
*/
#include <DFRobot_VL6180X.h>
DFRobot_VL6180X VL6180X;
uint8_t flag = 0;
uint8_t ret= 1;
void interrupt()
{
if(flag ==0){
flag = 1;
}
}
void setup() {
Serial.begin(9600);
while(!(VL6180X.begin())){
Serial.println("Please check that the IIC device is properly connected!");
delay(1000);
}
/** 开启INT引脚的通知功能
* mode:
* VL6180X_DIS_INTERRUPT 不开启中断
* VL6180X_LOW_INTERRUPT 开启中断,INT引脚默认输出低电平
* VL6180X_HIGH_INTERRUPT 开启中断,INT引脚默认输出高电平
* 注意:当使用VL6180X_LOW_INTERRUPT模式开启中断时,请用“RISING”来触发中断,当使用VL6180X_HIGH_INTERRUPT模式开启中断时,请用“FALLING”来触发中断。
*/
VL6180X.setInterrupt(/*mode*/VL6180X_HIGH_INTERRUPT);
/** 配置采集环境光的中断模式
* mode
* interrupt disable : VL6180X_INT_DISABLE 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
VL6180X.alsConfigInterrupt(/*mode*/VL6180X_NEW_SAMPLE_READY);
/** 配置测距的中断模式
* mode
* interrupt disable : VL6180X_INT_DISABLE 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
VL6180X.rangeConfigInterrupt(VL6180X_NEW_SAMPLE_READY);
/*配置环境光采集周期*/
VL6180X.alsSetInterMeasurementPeriod(/* periodMs 0-25500ms */1000);
#if defined(ESP32) || defined(ESP8266)||defined(ARDUINO_SAM_ZERO)
attachInterrupt(digitalPinToInterrupt(D9)/*Query the interrupt number of the D9 pin*/,interrupt,FALLING);
#else
/* The Correspondence Table of AVR Series Arduino Interrupt Pins And Terminal Numbers
* ---------------------------------------------------------------------------------------
* | | DigitalPin | 2 | 3 | |
* | Uno, Nano, Mini, other 328-based |--------------------------------------------|
* | | Interrupt No | 0 | 1 | |
* |-------------------------------------------------------------------------------------|
* | | Pin | 2 | 3 | 21 | 20 | 19 | 18 |
* | Mega2560 |--------------------------------------------|
* | | Interrupt No | 0 | 1 | 2 | 3 | 4 | 5 |
* |-------------------------------------------------------------------------------------|
* | | Pin | 3 | 2 | 0 | 1 | 7 | |
* | Leonardo, other 32u4-based |--------------------------------------------|
* | | Interrupt No | 0 | 1 | 2 | 3 | 4 | |
* |--------------------------------------------------------------------------------------
*/
/* The Correspondence Table of micro:bit Interrupt Pins And Terminal Numbers
* ---------------------------------------------------------------------------------------------------------------------------------------------
* | micro:bit | DigitalPin |P0-P20 can be used as an external interrupt |
* | (When using as an external interrupt, |---------------------------------------------------------------------------------------------|
* |no need to set it to input mode with pinMode)|Interrupt No|Interrupt number is a pin digital value, such as P0 interrupt number 0, P1 is 1 |
* |-------------------------------------------------------------------------------------------------------------------------------------------|
*/
attachInterrupt(/*Interrupt No*/0,interrupt,FALLING);//Open the external interrupt 0, connect INT1/2 to the digital pin of the main control:
//UNO(2), Mega2560(2), Leonardo(3), microbit(P0).
#endif
/*开启交叉测量模式*/
VL6180X.startInterleavedMode();
}
void loop() {
if(flag == 1){
/** 读取测量环境光的中断状态
* 返回值与设置的中断模式相对应
* No threshold events reported : 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
if(VL6180X.alsGetInterruptStatus() == VL6180X_NEW_SAMPLE_READY){
/*获得采集的环境光数据*/
float lux = VL6180X.alsGetMeasurement();
/*清除因采集环境光而产生的中断*/
VL6180X.clearAlsInterrupt();
String str ="ALS: "+String(lux)+" lux";
Serial.println(str);
flag = 0;
}
/** 读取测距中断状态
* 返回值与设置的中断模式相对应
* No threshold events reported : 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
if(VL6180X.rangeGetInterruptStatus() == VL6180X_NEW_SAMPLE_READY){
flag = 0;
/*获得测量的距离数据*/
uint8_t range = VL6180X.rangeGetMeasurement();
/*获得范围值的判断结果*/
uint8_t status = VL6180X.getRangeResult();
/*清除因测量距离而产生的中断*/
VL6180X.clearRangeInterrupt();
String str1 = "Range: "+String(range) + " mm";
switch(status){
case VL6180X_NO_ERR:
Serial.println(str1);
break;
case VL6180X_EARLY_CONV_ERR:
Serial.println("RANGE ERR: ECE check failed !");
break;
case VL6180X_MAX_CONV_ERR:
Serial.println("RANGE ERR: System did not converge before the specified max!");
break;
case VL6180X_IGNORE_ERR:
Serial.println("RANGE ERR: Ignore threshold check failed !");
break;
case VL6180X_MAX_S_N_ERR:
Serial.println("RANGE ERR: Measurement invalidated!");
break;
case VL6180X_RAW_Range_UNDERFLOW_ERR:
Serial.println("RANGE ERR: RESULT_RANGE_RAW < 0!");
break;
case VL6180X_RAW_Range_OVERFLOW_ERR:
Serial.println("RESULT_RANGE_RAW is out of range !");
break;
case VL6180X_Range_UNDERFLOW_ERR:
Serial.println("RANGE ERR: RESULT__RANGE_VAL < 0 !");
break;
case VL6180X_Range_OVERFLOW_ERR:
Serial.println("RANGE ERR: RESULT__RANGE_VAL is out of range !");
break;
default:
Serial.println("RANGE ERR: Systerm err!");
break;
}
}
}
}
结果
样例代码3 - 设置距离阈值中断
烧录代码,打开串口,当距离超出设置阈值是串口会打印出数据。
/**
* @file rangeContinuousInterruptMode.ino
* @brief 本传感器工作可工作在四种中断模式下,分别是低于下阈值触发中断模式、高于上阈值触发中断模式、低于下阈值或者高于上阈值触发中断模式以及新样本值采集完成触发中断模式
* @n 本示例介绍了在连续测量范围模式下的四种中断
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [yangfeng]<feng.yang@dfrobot.com>
* @version V1.0
* @date 2021-03-08
* @get from https://www.dfrobot.com
* @url https://github.com/DFRobot/DFRobot_VL6180X
*/
#include <DFRobot_VL6180X.h>
DFRobot_VL6180X VL6180X;
uint8_t ret= 1;
uint8_t flag = 0;
void interrupt()
{
if(flag ==0){
flag = 1;
}
}
void setup() {
Serial.begin(9600);
while(!(VL6180X.begin())){
Serial.println("Please check that the IIC device is properly connected!");
delay(1000);
}
/** 开启INT引脚的通知功能
* mode:
* VL6180X_DIS_INTERRUPT 不开启中断
* VL6180X_LOW_INTERRUPT 开启中断,INT引脚默认输出低电平
* VL6180X_HIGH_INTERRUPT 开启中断,INT引脚默认输出高电平
* 注意:当使用VL6180X_LOW_INTERRUPT模式开启中断时,请用“RISING”来触发中断,当使用VL6180X_HIGH_INTERRUPT模式开启中断时,请用“FALLING”来触发中断。
*/
VL6180X.setInterrupt(/*mode*/VL6180X_HIGH_INTERRUPT);
/** 配置测距的中断模式
* mode
* interrupt disable : VL6180X_INT_DISABLE 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
VL6180X.rangeConfigInterrupt(VL6180X_OUT_OF_WINDOW);
/*设置测距周期*/
VL6180X.rangeSetInterMeasurementPeriod(/* periodMs 0-25500ms */1000);
/*设置阈值*/
VL6180X.setRangeThresholdValue(/*thresholdL 0-255mm */40,/*thresholdH 0-255mm*/100);
#if defined(ESP32) || defined(ESP8266)||defined(ARDUINO_SAM_ZERO)
attachInterrupt(digitalPinToInterrupt(D9)/*Query the interrupt number of the D9 pin*/,interrupt,FALLING);
#else
/* The Correspondence Table of AVR Series Arduino Interrupt Pins And Terminal Numbers
* ---------------------------------------------------------------------------------------
* | | DigitalPin | 2 | 3 | |
* | Uno, Nano, Mini, other 328-based |--------------------------------------------|
* | | Interrupt No | 0 | 1 | |
* |-------------------------------------------------------------------------------------|
* | | Pin | 2 | 3 | 21 | 20 | 19 | 18 |
* | Mega2560 |--------------------------------------------|
* | | Interrupt No | 0 | 1 | 2 | 3 | 4 | 5 |
* |-------------------------------------------------------------------------------------|
* | | Pin | 3 | 2 | 0 | 1 | 7 | |
* | Leonardo, other 32u4-based |--------------------------------------------|
* | | Interrupt No | 0 | 1 | 2 | 3 | 4 | |
* |--------------------------------------------------------------------------------------
*/
/* The Correspondence Table of micro:bit Interrupt Pins And Terminal Numbers
* ---------------------------------------------------------------------------------------------------------------------------------------------
* | micro:bit | DigitalPin |P0-P20 can be used as an external interrupt |
* | (When using as an external interrupt, |---------------------------------------------------------------------------------------------|
* |no need to set it to input mode with pinMode)|Interrupt No|Interrupt number is a pin digital value, such as P0 interrupt number 0, P1 is 1 |
* |-------------------------------------------------------------------------------------------------------------------------------------------|
*/
attachInterrupt(/*Interrupt No*/0,interrupt,FALLING);//Open the external interrupt 0, connect INT1/2 to the digital pin of the main control:
//UNO(2), Mega2560(2), Leonardo(3), microbit(P0).
#endif
/*开始连续测距模式*/
VL6180X.rangeStartContinuousMode();
}
void loop() {
if(flag == 1){
flag = 0;
/**读取并判断产生的中断是否与设置中断相同
* No threshold events reported : 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
if(VL6180X.rangeGetInterruptStatus() == VL6180X_OUT_OF_WINDOW){
/*获得测量的距离数据*/
uint8_t range = VL6180X.rangeGetMeasurement();
/*获得范围值的判断结果*/
uint8_t status = VL6180X.getRangeResult();
/*清除因测量距离而产生的中断*/
VL6180X.clearRangeInterrupt();
String str1 = "Range: "+String(range) + " mm";
switch(status){
case VL6180X_NO_ERR:
Serial.println(str1);
break;
case VL6180X_EARLY_CONV_ERR:
Serial.println("RANGE ERR: ECE check failed !");
break;
case VL6180X_MAX_CONV_ERR:
Serial.println("RANGE ERR: System did not converge before the specified max!");
break;
case VL6180X_IGNORE_ERR:
Serial.println("RANGE ERR: Ignore threshold check failed !");
break;
case VL6180X_MAX_S_N_ERR:
Serial.println("RANGE ERR: Measurement invalidated!");
break;
case VL6180X_RAW_Range_UNDERFLOW_ERR:
Serial.println("RANGE ERR: RESULT_RANGE_RAW < 0!");
break;
case VL6180X_RAW_Range_OVERFLOW_ERR:
Serial.println("RESULT_RANGE_RAW is out of range !");
break;
case VL6180X_Range_UNDERFLOW_ERR:
Serial.println("RANGE ERR: RESULT__RANGE_VAL < 0 !");
break;
case VL6180X_Range_OVERFLOW_ERR:
Serial.println("RANGE ERR: RESULT__RANGE_VAL is out of range !");
break;
default:
Serial.println("RANGE ERR: Systerm err!");
break;
}
}
}
}
结果
样例代码4 - 设置环境光阈值中断
烧录代码,打开串口,当环境光超出设置阈值是串口会打印出数据。
/**
* @file alsContinuousInterruptMode.ino
* @brief 本传感器工作可工作在四种中断模式下,分别是低于下阈值触发中断模式、高于上阈值触发中断模式、低于下阈值或者高于上阈值触发中断模式以及新样本值采集完成触发中断模式
* @n 本示例介绍了在连续测量环境光模式下的四种中断
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @licence The MIT License (MIT)
* @author [yangfeng]<feng.yang@dfrobot.com>
* @version V1.0
* @date 2021-03-08
* @get from https://www.dfrobot.com
* @url https://github.com/DFRobot/DFRobot_VL6180X
*/
#include <DFRobot_VL6180X.h>
DFRobot_VL6180X VL6180X;
uint8_t flag = 0;
uint8_t ret= 1;
void interrupt()
{
if(flag ==0){
flag = 1;
}
}
void setup() {
Serial.begin(9600);
while(!(VL6180X.begin())){
Serial.println("Please check that the IIC device is properly connected!");
delay(1000);
}
/*配置环境光采集周期*/
VL6180X.alsSetInterMeasurementPeriod(/* periodMs 0-25500ms */1000);
/** 开启INT引脚的通知功能
* mode:
* VL6180X_DIS_INTERRUPT 不开启中断
* VL6180X_LOW_INTERRUPT 开启中断,INT引脚默认输出低电平
* VL6180X_HIGH_INTERRUPT 开启中断,INT引脚默认输出高电平
* 注意:当使用VL6180X_LOW_INTERRUPT模式开启中断时,请用“RISING”来触发中断,当使用VL6180X_HIGH_INTERRUPT模式开启中断时,请用“FALLING”来触发中断。
*/
VL6180X.setInterrupt(/*mode*/VL6180X_LOW_INTERRUPT);
/** 配置采集环境光的中断模式
* mode
* interrupt disable : VL6180X_INT_DISABLE 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
VL6180X.alsConfigInterrupt(/*mode*/VL6180X_OUT_OF_WINDOW);
/**设置采集增益
* gain:
* 20 times gain: VL6180X_ALS_GAIN_20
* 10 times gain: VL6180X_ALS_GAIN_10
* 5 times gain: VL6180X_ALS_GAIN_5
* 2.5 times gain: VL6180X_ALS_GAIN_2_5
* 1.57 times gain: VL6180X_ALS_GAIN_1_67
* 1.27 times gain: VL6180X_ALS_GAIN_1_25
* 1 times gain: VL6180X_ALS_GAIN_1
* 40 times gain: VL6180X_ALS_GAIN_40
*/
//VL6180X.setALSGain(VL6180X_ALS_GAIN_1);
//这里设置阈值的接口和设置增益的接口相关联,若要同时指定增益和阈值,请先设置增益,再设置阈值
VL6180X.setALSThresholdValue(/*thresholdL 0-65535lux */40,/*thresholdH 0-65535lux*/100);
#if defined(ESP32) || defined(ESP8266)||defined(ARDUINO_SAM_ZERO)
attachInterrupt(digitalPinToInterrupt(D9)/*Query the interrupt number of the D9 pin*/,interrupt,RISING);
#else
/* The Correspondence Table of AVR Series Arduino Interrupt Pins And Terminal Numbers
* ---------------------------------------------------------------------------------------
* | | DigitalPin | 2 | 3 | |
* | Uno, Nano, Mini, other 328-based |--------------------------------------------|
* | | Interrupt No | 0 | 1 | |
* |-------------------------------------------------------------------------------------|
* | | Pin | 2 | 3 | 21 | 20 | 19 | 18 |
* | Mega2560 |--------------------------------------------|
* | | Interrupt No | 0 | 1 | 2 | 3 | 4 | 5 |
* |-------------------------------------------------------------------------------------|
* | | Pin | 3 | 2 | 0 | 1 | 7 | |
* | Leonardo, other 32u4-based |--------------------------------------------|
* | | Interrupt No | 0 | 1 | 2 | 3 | 4 | |
* |--------------------------------------------------------------------------------------
*/
/* The Correspondence Table of micro:bit Interrupt Pins And Terminal Numbers
* ---------------------------------------------------------------------------------------------------------------------------------------------
* | micro:bit | DigitalPin |P0-P20 can be used as an external interrupt |
* | (When using as an external interrupt, |---------------------------------------------------------------------------------------------|
* |no need to set it to input mode with pinMode)|Interrupt No|Interrupt number is a pin digital value, such as P0 interrupt number 0, P1 is 1 |
* |-------------------------------------------------------------------------------------------------------------------------------------------|
*/
attachInterrupt(/*Interrupt No*/0,interrupt,RISING);//Open the external interrupt 0, connect INT1/2 to the digital pin of the main control:
//UNO(2), Mega2560(2), Leonardo(3), microbit(P0).
#endif
/*开启连续采集模式*/
VL6180X.alsStartContinuousMode();
}
void loop() {
if(flag == 1){
flag = 0;
/**读取并判断产生的中断是否与设置中断相同
* interrupt disable : VL6180X_INT_DISABLE 0
* value < thresh_low : VL6180X_LEVEL_LOW 1
* value > thresh_high: VL6180X_LEVEL_HIGH 2
* value < thresh_low OR value > thresh_high: VL6180X_OUT_OF_WINDOW 3
* new sample ready : VL6180X_NEW_SAMPLE_READY 4
*/
if(VL6180X.alsGetInterruptStatus() == VL6180X_OUT_OF_WINDOW){
/*获得采集数据*/
float lux = VL6180X.alsGetMeasurement();
/*清除中断*/
VL6180X.clearAlsInterrupt();
String str ="ALS: "+String(lux)+" lux";
Serial.println(str);
}
}
}
结果
常见问题
还没有客户对此产品有任何问题,欢迎通过qq或者论坛联系我们!
更多问题及有趣的应用,可以 访问论坛 进行查阅或发帖。