简介
这是一款开放式双探头超声波测距模块,通信方式为I2C,采用Gravity标准PH2.0-4P立式贴片座接口。该模块兼容arduino、树莓派等各种3.3V或5V逻辑电平的主控板。模块自带温度补偿,平整墙面的有效测距量程为2–500cm,分辨率为1cm,误差约为±1%。 设计150cm、300cm、500cm 三个测距量程可供程序选择切换,更短的量程设置会带来更短的测距周期和更低的测距灵敏度,用户可根据实际使用需求灵活设置。
技术规格
- 供电电压:3.3V~5.5V DC
- 工作电流:20mA
- 工作温度范围:-10℃~+70℃
- 有效测距量程:2cm~500cm(可设置,最大量程500CM)
- 分辨率:1cm
- 精度:1%
- 方向角:60°
- 测量频率:50Hz Max
- 模块尺寸:47mm × 22 mm
接口说明
引脚 | 引脚说明 |
---|---|
VCC | 电源输入(3.3V-5.5V) |
GND | 电源地 |
C | I2C时钟线SCL |
D | I2C数据线SDA |
使用教程
URM09是一款简洁实用的超声波传感器,它采用的是I2C通信方式,可以方便的与具有I2C接口的主板时行通信
寄存器表
URM09 Ultrasonic Sensor(Gravity I²C)(V2.0)寄存器一览表
寄存器表
URM09 Ultrasonic Sensor(Gravity I²C)(V3.0)寄存器一览表
寄存器(8bit) | 名称 | 读写 | 数据范围 | 默认值 | 描述 |
---|---|---|---|---|---|
0x00 | 设备地址 | R/W | 0x08-0x77 | 0x11 | 模块的设备地址 重启后生效 |
0x01 | 产品ID | R | 0x01 | 用于产品校验 | |
0x02 | 版本号 | R | 0x10 | 用于版本校验(0x10表示V1.0) | |
0x03 0x04 |
距离值高位 距离值低位 |
R R |
0x00 0xFF |
0x00 0x00 |
LSB表示1cm 例:0x64代表100cm |
0x05 0x06 |
温度值高位 温度值低位 |
R R |
0x00 0xFF |
0x00 0x00 |
实际温度扩大10倍后的值 例:读出的值为0x00fe,则实际温度是0x00fe/10=25.4℃ |
0x07 | 配置寄存器 | R/W | 0x00 | bit7(距离测量模式控制位): 0:被动测量,发送一次测距命令,模块测量一次距离并将测量的距离值存入距离寄存器 1:自动测量模式,模块一直在进行距离测量,并不断更新距离寄存器 |
|
0x08 | 命令寄存器 | R/W | 0x00 | 被动模式下写0x01到该寄存器,模块进行一次测距,自动测量模式下写入数据无效 |
注意:新版本已经取消了程序选择切换150cm、300cm、500cm的测距范围,并对测距方案进行了优化,以提高产品的稳定性和探头的兼容性。因此,尽管库中的代码未做相应修改,但使用软件程序来配置测距范围不会产生效果。
硬件连接
将模块通过I2C接口与UNO连接,如图:
Arduino 距离及温度测量
模块出厂默认为被动测量模式,通过写命令寄存器发送测距命令,模块收到命令后便启动一次测距(测距时间与测距量程有关),当测距完成后,读取距离寄存器便可得到距离值。而温度测量直接读出温度寄存器后,经过简单处理就可以得到测得的温度值。
演示代码
/*!
* @file PassiveMesure.ino
* @brief Passive measurement of distance and temperature
* @n This example is the ultrasonic passive measurement of distance and module temperature and printing on the serial port
* @copyright Copyright (c) 2010 DFRobot Co.Ltd (http://www.dfrobot.com)
* @license The MIT License (MIT)
* @author ZhixinLiu(zhixin.liu@dfrobot.com)
* @version version V1.2
* @date 2020-9-30
* @url https://github.com/DFRobot/DFRobot_URM09
*/
#include "DFRobot_URM09.h"
/* Create a URM09 object to communicate with IIC. */
DFRobot_URM09 URM09;
void setup() {
Serial.begin(115200);
/**
* I2c device number 1-127
* When the i2c device number is not passed, the default parameter is 0x11
*/
while(!URM09.begin()){
Serial.println("I2C device number error ");
delay(1000);
}
/**
* The module is configured in automatic mode or passive
* MEASURE_MODE_AUTOMATIC automatic mode
* MEASURE_MODE_PASSIVE passive mode
* The measurement distance is set to 500,300,150
* MEASURE_RANG_500 Ranging from 500
* MEASURE_RANG_300 Ranging from 300
* MEASURE_RANG_150 Ranging from 150
*/
URM09.setModeRange(MEASURE_MODE_PASSIVE ,MEASURE_RANG_500);
delay(100);
}
void loop() {
URM09.measurement(); // Send ranging command
delay(100);
int16_t dist = URM09.getDistance(); // Read distance
float temp = URM09.getTemperature(); // Read temperature
Serial.print(dist, DEC); Serial.print(" cm------");
Serial.print(temp, 1); Serial.println(" C");
delay(100);
}
演示效果
Raspberry 距离及温度测量
演示代码
- 下载URM09库文件
# -*- coding:utf-8 -*-
'''
# PassiveMeasure.py
#
# Connect board with raspberryPi.
# Make board power and URM09 connection correct.
# Run this demo.
#
# Set the i2c communication device number.
# Set test mode and distance.
# Get temperature and distance data.
#
# Copyright [DFRobot](http://www.dfrobot.com), 2016
# Copyright GNU Lesser General Public License
#
# version V2.0
# date 2019-7-09
'''
import sys
import time
import URM09
sys.path.append("../..")
''' Create a URM09 object to communicate with I2C. '''
URM09 = URM09.DFRobot_URM09()
''' Set the i2c device number '''
URM09.begin(0x11)
time.sleep(0.1)
'''
# The module is configured in automatic mode or passive
# _MEASURE_MODE_AUTOMATIC // automatic mode
# _MEASURE_MODE_PASSIVE // passive mode
# The measurement distance is set to 500,300,100
# _MEASURE_RANG_500 // Ranging from 500
# _MEASURE_RANG_300 // Ranging from 300
# _MEASURE_RANG_150 // Ranging from 100
'''
URM09.SetModeRange(URM09._MEASURE_MODE_PASSIVE, URM09._MEASURE_RANG_500)
while(1):
''' Write command register and send ranging command '''
URM09.SetMeasurement()
time.sleep(0.1)
''' Read distance register '''
dist = URM09.i2cReadDistance()
''' Read temperature register '''
temp = URM09.i2cReadTemperature()
print("Temperature is %.2f .c " % (temp / 10))
print("Distance is %d cm " % dist)
time.sleep(0.1)
演示结果
Mind+ Python模式编程(行空板)
Mind+Python模式为完整Python编程,因此需要能运行完整Python的主控板,此处以行空板为例说明
连接图
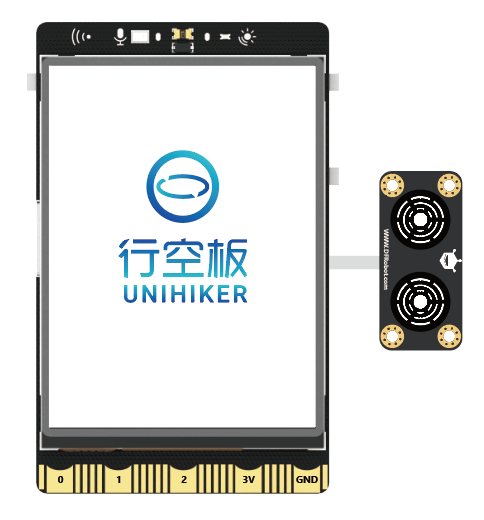
操作步骤
1、下载及安装官网最新软件。下载地址:https://www.mindplus.cc 详细教程:Mind+基础wiki教程-软件下载安装
2、切换到“Python模式”。“扩展”中选择“官方库”中的“行空板”和“pinpong库”中的”pinpong初始化“和“I2C超声波测距传感器”。切换模式和加载库的详细操作链接
3、进行编程
4、连接行空板,程序点击运行后,可在终端查看数据。行空板官方文档-行空板快速上手教程 (unihiker.com)
代码编程
以pinpong库为例,行空板官方文档-行空板快速上手教程 (unihiker.com)
# -*- coding: UTF-8 -*-
# MindPlus
# Python
from pinpong.libs.dfrobot_urm09 import URM09
from pinpong.board import Board
import time
Board().begin()
ult1 = URM09(17)
ult1.set_mode_range(ult1._MEASURE_MODE_AUTOMATIC, ult1._MEASURE_RANG_500)
while True:
print((str("距离:") + str(ult1.distance_cm())))
print((str("温度:") + str(ult1.temp_c())))
time.sleep(1)
探测角度及灵敏度说明
超声波传感器的物理特性决定了其实际具有不规则的探测区域,因此超声波测距传感器的探测角度难以被准确的定义。我们分别使用了2种参考目标障碍物对多样本产品进行了测试,对应目标的参照检测区域如下图示:
疑难解答
如果遇到技术问题,请登陆到我们的售后论坛留言,我们会尽快解答您的问题。
更多问题及有趣的应用,可以 访问论坛 进行查阅或发帖!