简介
想过要把黄浦江边正旦国际大厦的广告牌搬回家吗?32x16 RGB LED矩阵屏幕可以帮你实现这个愿望。这是一款全彩、高亮LED矩阵屏,常被用在一些广告墙和显示屏上。屏幕采用高分子防火PC塑胶面罩,具备高温阻燃特性和良好的散热性能。可以确保产品的强度和外形稳定性,高低温工作时均能保持模块之间不会相互挤压变形,使产品可以在恶劣环境下正常使用,底壳有便于安装的安装孔,面罩不反光,耐紫外线,不褪色.
该面板由512个RGB led按照32x16网格组成。背后,有两个IDC 连接器,一个输入接口,一个输出接口;1/8扫描驱动显示屏。面板需要用到13个IO 引脚(6个数据位,7个控制位),一个5V电压,2A以上输出电流的电源供电。
注意:此面板在供电和RAM足够的情况下,可以任意级联,组合成一个更大体积的灯板。当级联数量大于4之后,Arduino UNO的运算能力就稍显不足了,需要使用Mega2560,树莓派或者其他的设备来驱动这块RGB面板
技术规格
- 驱动电压:DC 4.8-5.5V
- 平均功耗:小于500W/㎡
- 最大功耗:小于1000w/㎡
- 分辨率:32*16=512 DOTS
- 可视角度水平:≧160°
- 控制方式:同步控制
- 换幁频率:≧60Hz
- 白平衡亮度:≧1200cd/㎡
- 刷新频率:≧300Hz
- 像素间距:6mm
- 模组尺寸:192mm*96mm
- 模组厚度:11mm
- 驱动方式:1/8扫描
- 平均无故障时间:≧5000小时
- 寿命:75000~100000小时
引脚说明
**注意:**IN-PUT和OUT-PUT引脚顺序一样,POWER电源供电5V
-
标号 | 名称 | 功能描述 |
---|---|---|
1 | DR1 | 高位R数据 |
2 | DG1 | 高位G数据 |
3 | DB1 | 高位B数据 |
4 | GND | 地 |
5 | DR2 | 低位R数据 |
6 | DG2 | 低位G数据 |
7 | DB2 | 低位B数据 |
8 | GND | 地 |
9 | A | A行选择 |
10 | B | B行选择 |
11 | C | C行选择 |
12 | NC | 接地 |
13 | CLK | 时钟 |
14 | STB | 闸门 |
15 | OE | 输出启用 |
16 | GND | 地 |
IN-PUT and OUT-PUT
标号 | 名称 | 功能描述 |
---|---|---|
1 | VCC | 5V电源 |
2 | VCC | 5V电源 |
3 | GND | 地 |
4 | GND | 地 |
POWER
使用教程
按照引脚说明连接好硬件,并下载样例代码到UNO中,即可看到漂亮的显示效果。
准备
- 硬件
- DFRduino UNO x1
- IO 传感器扩展板 V7.1 x1
- 杜邦线 若干
- 软件
- Arduino IDE, 点击下载Arduino IDE
接线图
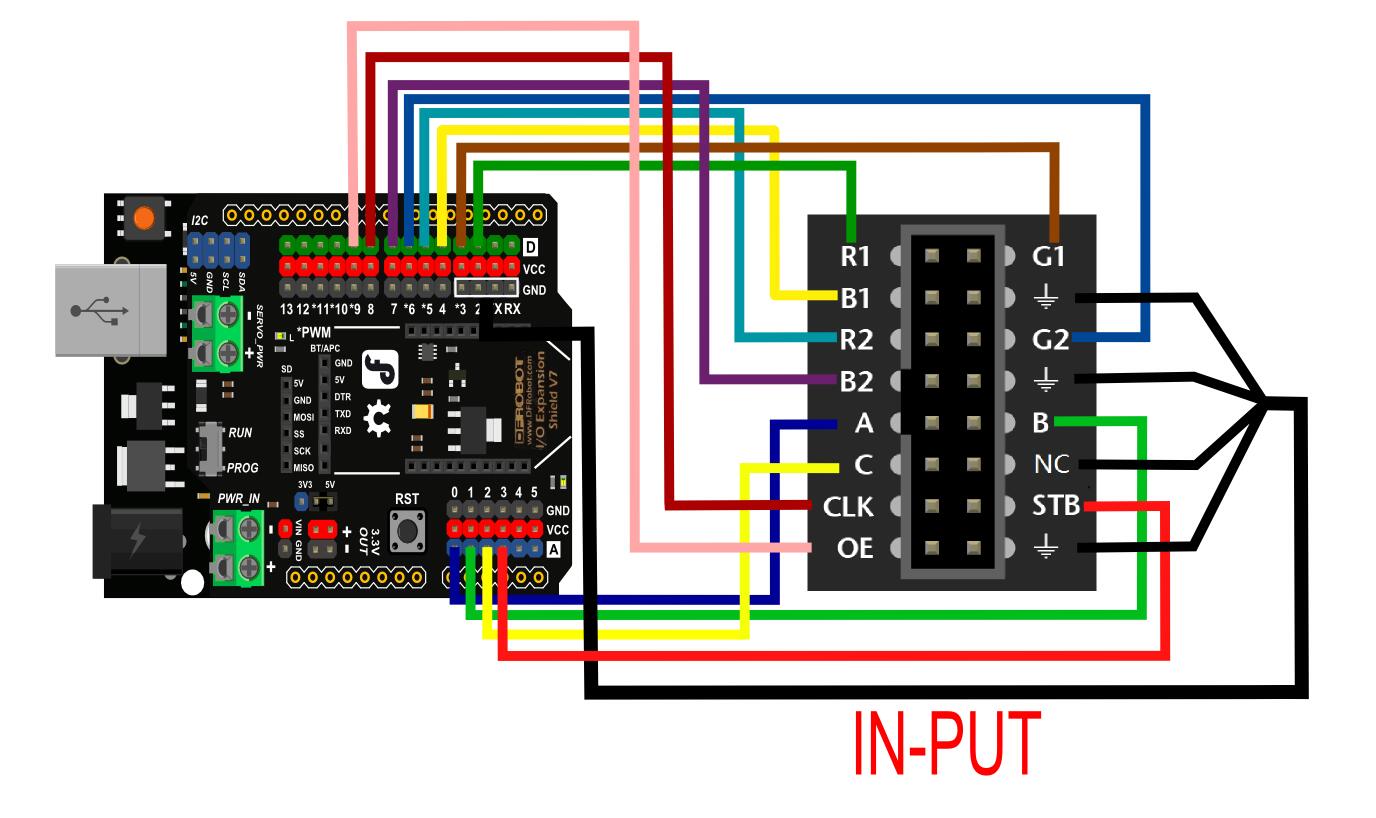
注意:灯板使用时需要外接供电,USB仅5V@500mA输出。
样例代码1
点击下载库文件Adafruit-GFX. RGB-matrix-Panel.Adafruit_BusIO.如何安装库?
/***************************************************
*
* For 32x16 RGB LED matrix.
*
* @author lg.gang
* @version V1.0
* @date 2016-10-28
*
* GNU Lesser General Public License.
* See <http://www.gnu.org/licenses/> for details.
* All above must be included in any redistribution
* ****************************************************/
#include <Adafruit_GFX.h> // Core graphics library
#include <RGBmatrixPanel.h> // Hardware-specific library
#define CLK 8 // MUST be on PORTB! (Use pin 11 on Mega)
#define LAT A3
#define OE 9
#define A A0
#define B A1
#define C A2
RGBmatrixPanel matrix(A, B, C, CLK, LAT, OE, false);
void setup() {
matrix.begin();
}
void loop() {
// draw a pixel in solid white
matrix.drawPixel(0, 0, matrix.Color333(7, 7, 7));
delay(500);
// fix the screen with green
matrix.fillRect(0, 0, 32, 16, matrix.Color333(0, 7, 0));
delay(500);
// draw a box in yellow
matrix.drawRect(0, 0, 32, 16, matrix.Color333(7, 7, 0));
delay(500);
// draw an 'X' in red
matrix.drawLine(0, 0, 31, 15, matrix.Color333(7, 0, 0));
matrix.drawLine(31, 0, 0, 15, matrix.Color333(7, 0, 0));
delay(500);
// draw a blue circle
matrix.drawCircle(7, 7, 7, matrix.Color333(0, 0, 7));
delay(500);
// fill a violet circle
matrix.fillCircle(23, 7, 7, matrix.Color333(7, 0, 7));
delay(500);
// fill the screen with 'black'
matrix.fillScreen(matrix.Color333(0, 0, 0));
// draw some text!
matrix.setCursor(1, 0); // start at top left, with one pixel of spacing
matrix.setTextSize(1); // size 1 == 8 pixels high
// print each letter with a rainbow color
matrix.setTextColor(matrix.Color333(7,0,0));
matrix.print('1');
matrix.setTextColor(matrix.Color333(7,4,0));
matrix.print('6');
matrix.setTextColor(matrix.Color333(7,7,0));
matrix.print('x');
matrix.setTextColor(matrix.Color333(4,7,0));
matrix.print('3');
matrix.setTextColor(matrix.Color333(0,7,0));
matrix.print('2');
matrix.setCursor(1, 9); // next line
matrix.setTextColor(matrix.Color333(0,7,7));
matrix.print('*');
matrix.setTextColor(matrix.Color333(0,4,7));
matrix.print('R');
matrix.setTextColor(matrix.Color333(0,0,7));
matrix.print('G');
matrix.setTextColor(matrix.Color333(4,0,7));
matrix.print('B');
matrix.setTextColor(matrix.Color333(7,0,4));
matrix.print("*");
delay(5000);
}
样例代码2
/***************************************************
*
* For 32x16 RGB LED matrix.
*
* @author lg.gang
* @version V1.0
* @date 2016-10-28
*
* GNU Lesser General Public License.
* See <http://www.gnu.org/licenses/> for details.
* All above must be included in any redistribution
* ****************************************************/
#include <Adafruit_GFX.h> // Core graphics library
#include <RGBmatrixPanel.h> // Hardware-specific library
// Similar to F(), but for PROGMEM string pointers rather than literals
#define F2(progmem_ptr) (const __FlashStringHelper *)progmem_ptr
#define CLK 8 // MUST be on PORTB! (Use pin 11 on Mega)
#define LAT A3
#define OE 9
#define A A0
#define B A1
#define C A2
// Last parameter = 'true' enables double-buffering, for flicker-free,
// buttery smooth animation. Note that NOTHING WILL SHOW ON THE DISPLAY
// until the first call to swapBuffers(). This is normal.
RGBmatrixPanel matrix(A, B, C, CLK, LAT, OE, false);
// Double-buffered mode consumes nearly all the RAM available on the
// Arduino Uno -- only a handful of free bytes remain. Even the
// following string needs to go in PROGMEM:
const char str[] PROGMEM = "Welcome to DFrobot 32x16 RGB LED Matrix";
int textX = matrix.width(),
textMin = sizeof(str) * -12,
hue = 0;
int8_t ball[3][4] = {
{ 3, 0, 1, 1 }, // Initial X,Y pos & velocity for 3 bouncy balls
{ 17, 15, 1, -1 },
{ 27, 4, -1, 1 }
};
static const uint16_t PROGMEM ballcolor[3] = {
0x0080, // Green=1
0x0002, // Blue=1
0x1000 // Red=1
};
void setup() {
matrix.begin();
matrix.setTextWrap(false); // Allow text to run off right edge
matrix.setTextSize(2);
}
void loop() {
byte i;
// Clear background
matrix.fillScreen(0);
// Bounce three balls around
for(i=0; i<3; i++) {
// Draw 'ball'
matrix.fillCircle(ball[i][0], ball[i][1], 5, pgm_read_word(&ballcolor[i]));
// Update X, Y position
ball[i][0] += ball[i][2];
ball[i][1] += ball[i][3];
// Bounce off edges
if((ball[i][0] == 0) || (ball[i][0] == (matrix.width() - 1)))
ball[i][2] *= -1;
if((ball[i][1] == 0) || (ball[i][1] == (matrix.height() - 1)))
ball[i][3] *= -1;
}
// Draw big scrolly text on top
matrix.setTextColor(matrix.ColorHSV(hue, 255, 255, true));
matrix.setCursor(textX, 1);
matrix.print(F2(str));
// Move text left (w/wrap), increase hue
if((--textX) < textMin) textX = matrix.width();
hue += 7;
if(hue >= 1536) hue -= 1536;
// Update display
matrix.swapBuffers(false);
}
结果
样列代码1:LED模组会轮流显示:一个白点、全屏绿色、一个黄色的矩形框、一个红色的X、一个蓝色的圆、一个填充紫圆、英文字。
样列代码2:LED模组会从右往左显示Welcome to DFrobot 32x16 RGB LED Matrix英文字和红绿蓝3个填充圆。
常见问题
还没有客户对此产品有任何问题,欢迎通过qq或者论坛联系我们!
更多问题及有趣的应用,可以 访问论坛 进行查阅或发帖。